The biggest Python topics of 2023 ›
Object-Oriented Programming in Python
This topic covers object-oriented programming in Python with a focus on classes, subclassing, and dataclasses. It delves into inheritance, metaclasses, and SOLID principles to improve object-oriented design. The documents explore concepts like getter and setter methods, global variables, and how to leverage the power of classes for building complex systems in Python.
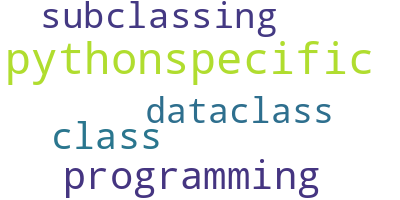
Python Classes: The Power of Object-Oriented Programming Article
In this tutorial, you’ll learn how to create and use full-featured classes in your Python code. Classes provide a great way to solve complex programming problems by approaching them through models that represent real-world objects.
https://realpython.com/python-classes/
SOLID Principles: Improve Object-Oriented Design in Python Article
In this tutorial, you’ll learn about the SOLID principles, which are five well-established standards for improving your object-oriented design in Python. By applying these principles, you can create object-oriented code that is more maintainable, extensible, scalable, and testable.
https://realpython.com/solid-principles-python/
Python Basics Exercises: Object-Oriented Programming Article
In this Python Basics Exercises course, you’ll review OOP, or object-oriented programming. You’ll practice creating classes, using classes to create new objects, and instantiating classes with attributes.
https://realpython.com/courses/object-oriented-programming-exercises/
Single and Double Underscores in Python Names Article
In this tutorial, you’ll learn a few Python naming conventions involving single and double underscores (_). You’ll learn how to use this character to differentiate between public and non-public names in APIs, write safe classes for subclassing purposes, avoid name clashes, and more.
https://realpython.com/python-double-underscore/
Design and Guidance: Object-Oriented Programming in Python Article
In this video course, you’ll learn about the SOLID principles, which are five well-established standards for improving your object-oriented design in Python. By applying these principles, you can create object-oriented code that is more maintainable, extensible, scalable, and testable.
https://realpython.com/courses/solid-principles-python/
What Does if __name__ == "__main__" Mean in Python? Article
In this video course, you’ll learn all about Python’s name-main idiom. You’ll learn what it does in Python, how it works, when to use it, when to avoid it, and how to refer to it.
https://realpython.com/courses/if-name-main-python/
Class Concepts: Object-Oriented Programming in Python Article
Python uses object-oriented programming to group data and associated operations together into classes. In this video course, you’ll learn how to write object-oriented code with classes, attributes, and methods.
https://realpython.com/courses/python-class-object/
Python Basics: Building Systems With Classes Article
In this video course, you’ll learn how to work with classes to build complex systems in Python. By composing classes, inheriting from other classes, and overriding class behavior, you’ll harness the power of object-oriented programming (OOP).
https://realpython.com/courses/python-basics-class/
Using and Creating Global Variables in Your Python Functions Article
In this tutorial, you’ll learn how to use global variables in Python functions using the global keyword or the built-in globals() function. You’ll also learn a few strategies to avoid relying on global variables because they can lead to code that’s difficult to understand, debug, and maintain.
https://realpython.com/python-use-global-variable-in-function/
Python Variables: Namespaces and Variable Scope Article
This post is a comprehensive guide on namespaces and variable scope. Learn about the four different name spaces and how to access each.
https://muhammadraza.me/2023/Python-Namespace/
Understanding Python Metaclasses Article
Metaclasses are part of the darker corners of Python and many developers avoid them. This article dives deep into how you can use them to reduce boilerplate code and build APIs.
https://blog.ionelmc.ro/2015/02/09/understanding-python-metaclasses/
Should You Write a class Without @dataclass? Article
This article makes the argument that you should always use @dataclass for building classes in Python. Read on to understand why, and maybe respond to Glyph’s call-to-action to tell him if you think he’s wrong.
https://blog.glyph.im/2023/02/data-classification.html
Python-Specific Design Patterns Article
This is a third article in a series on design patterns in Python, with this one talking about a variation on singletons, a pattern that uses dynamic function binding, and sentinels.
https://stackabuse.com/python-specific-design-patterns/
Inheritance and Internals: Object-Oriented Coding in Python Article
In this video course, you’ll learn about the various types of inheritance that you can use to write object-oriented code in Python. These include class inheritance, multilevel inheritance, and multiple inheritance, along with special methods and abstract base classes.
https://realpython.com/courses/python-class-inheritance/
Creating a Context Manager in Python Article
Objects with __enter__ and __exit__ methods can be used as context managers in Python. This article (and screencast) explains most of what you’ll want to know when creating your own context managers.
https://www.pythonmorsels.com/creating-a-context-manager/
Metaclasses in Python Article
Metaclasses are an important but mysterious behind-the-scenes mechanism for instantiating classes in Python. In this video course, you’ll learn how Python’s metaclasses work in object-oriented programming.
https://realpython.com/courses/python-metaclasses/
Unravelling global Article
Dive deep into global, how it works, its relationship with builtins and the namespace differences in Python.
https://snarky.ca/unravelling-global/
Use Properties to Add Dynamic Behavior to Attributes Article
This article teaches you how to use properties to add dynamic behavior to your attributes. It covers the most common use cases for properties, how to use them, and shows examples of real-world usages of this feature.
https://mathspp.com/blog/pydonts/properties
Getters and Setters in Python Article
In this video course, you’ll learn what getter and setter methods are, how Python properties are preferred over getters and setters when dealing with attribute access and mutation, and when to use getter and setter methods instead of properties in Python.
https://realpython.com/courses/getters-and-setters-python/
Dependency Injection in Python Article
“Dependency Injection (DI) is a design pattern that encourages loose coupling, maintainability, and testability within software applications.” Though more often associated with statically typed languages, the pattern can be applied with Python.
https://itnext.io/dependency-injection-in-python-a1e56ab8bdd0
State Patterns in Python Article
Design patterns are conventional solutions to common challenges in software development. The State Machine pattern encapsulates the flow of change in a system. Learn to implement state patterns and finite state machines in Python.
https://auth0.com/blog/state-pattern-in-python/