The biggest Python topics of 2023 ›
Python Error Handling and Debugging Best Practices
Exploring Python error handling and debugging techniques. This topic covers various aspects such as hiding stack traces in production, using assert for debugging and testing, raising exceptions, catching multiple exceptions, error handling best practices, and different approaches to error culture including tracking dependencies for function calls. The documents provide insights into efficiently handling errors, improving code reliability, and understanding different error-handling mechanisms in Python apps.
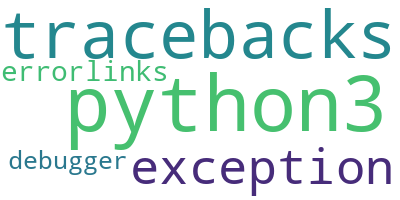
Use Unittest’s subtest Helper Article
When iterating over multiple arguments in testing a function, the subtest feature of the unittest module helps you track which part failed.
https://www.b-list.org/weblog/2023/dec/09/python-unittest-subtest/
Python Errors as Values Article
Error handling can be done in a variety of ways, and this article discusses why one organization decided to use returned error values instead of exceptions. Along the way, you’ll see comparisons between Python, Go, and Rust to better understand the different mechanisms.
https://www.inngest.com/blog/python-errors-as-values
The Categories of Bugs in Python Apps Article
This article categorizes errors in your Python code into three groups: type-checking, import explosions, and runtime errors. Learn how each is treated differently and how to better handle them in your programs.
https://threeofwands.com/the-types-of-errors-in-python-apps/
How to Catch Multiple Exceptions in Python Article
In this how-to tutorial, you’ll learn different ways of catching multiple Python exceptions. You’ll review the standard way of using a tuple in the except clause, but also expand your knowledge by exploring some other techniques, such as suppressing exceptions and using exception groups.
https://realpython.com/python-catch-multiple-exceptions/
Let’s Create a Python Debugger Together Article
Ever wondered how a debugger works? Implementing a simple one requires less code than you might think. Read on to find out how.
https://mostlynerdless.de/blog/2023/09/20/lets-create-a-python-debugger-together-part-1/
perf Engineering With Python 3.12 Article
Python 3.12 can run in a special mode that allows Python functions to appear in the output of the Linux perf profiler. This article walks you through a dummy application and using the profiler to find the bad parts.
https://www.petermcconnell.com/posts/perf_eng_with_py12/
Python’s raise: Raising Exceptions in Your Code Article
In this tutorial, you’ll learn how to raise exceptions in Python, which will improve your ability to efficiently handle errors and exceptional situations in your code. This way, you’ll write more reliable, robust, and maintainable code.
https://realpython.com/python-raise-exception/
Error Culture Article
This multi-part post talks about how getting notified about all errors leads to error fatigue from false positives and a culture where errors get ignored. Long term this can have disastrous consequences.
https://www.ryancheley.com/2023/10/29/error-culture/
FunctionTrace: Human-Oriented Profiling for Python Article
https://functiontrace.com/
Introducing flake8-logging Article
The Python standard library’s logging module is a go-to for adding observability to applications, but there are right and wrong ways to use it. This article is about a new linter that explicitly looks for problems with your logging calls.
https://adamj.eu/tech/2023/09/07/introducing-flake8-logging/
Why and How to Hide the Python Stack Trace Article
In production software you shouldn’t crash, but if you do, showing your users the stack trace can be disconcerting. This article covers how to hide the stack trace and what other information to provide.
https://www.bitecode.dev/p/why-and-how-to-hide-the-python-stack
Python: Profile a Section of Code With cProfile Article
“When trying to improve a slow function or module, it’s always a good idea to profile it. Here’s a snippet for quickly profiling a section of code with Python’s cProfile module.”
https://adamj.eu/tech/2023/07/23/python-profile-section-cprofile/
Avoiding Silent Failures: Best Practices for Error Handling Article
The Zen of Python famously states, “Errors should never pass silently.” But just what does that mean, and what should you do instead?
https://pybit.es/articles/python-errors-should-not-pass-silently/
Dependency Tracking for Python Function Calls Article
Tracking the code and data accessed by a function call can be used to draw dependency graphs, for debugging and profiling, and for cache invalidation. This article shows you a variety ways of doing it, as well as some initial ideas that don’t work very well.
https://amakelov.github.io/blog/deps/
Using Python’s assert to Debug and Test Your Code Article
In this course, you’ll learn how to use Python’s assert statement to document, debug, and test code in development. You’ll learn how assertions might be disabled in production code, so you shouldn’t use them to validate data. You’ll also learn about a few common pitfalls of assertions in Python.
https://realpython.com/courses/python-assert-statement/
Python Assertions, or Checking if a Cat Is a Dog Article
The articles explains the rules of using assertions in Python, and when not to use them. Includes details on the __debug__ object.
https://medium.com/towards-data-science/python-assertions-or-checking-if-a-cat-is-a-dog-ce11c55d143
pylyzer: A Fast Static Code Analyzer for Python Project
A fast static code analyzer & language server for Python
https://github.com/mtshiba/pylyzer
pystack: Inspect Stack Frames in Running or Crashed Python Project Started in 2023
🔍 🐍 Like pstack but for Python!
https://github.com/bloomberg/pystack
pymg: A Better Display for Stack Traces Project Started in 2023
pymg is a CLI that can interpret Python files by the Python interpreter and display the error message in a more readable way if an exception occurs.
https://github.com/mimseyedi/pymg
error-links: Add Links to Search Google to Your Tracebacks Project Started in 2023
Add Google and Python documentation links to the bottom of exceptions.
https://github.com/rodrigogiraoserrao/error-links
tracemem: Memory Tracker for Python Session Project Started in 2023
A lightweight tool to measure the full memory of a Python session
https://github.com/nyggus/tracemem