The biggest Python topics of 2023 ›
Python Type Hinting and Static Typing
The intersection of typechecking, typehinting, and compiler technologies in Python is explored through articles discussing the adoption of static typing in Python, the benefits of type hints for code readability and maintainability, and the use of static type checkers like Pyright and Mypy. The evolving landscape of Python as it incorporates more static typing features, such as type variables and typed dictionaries in Python 3.12, is also a key focus of the topic.
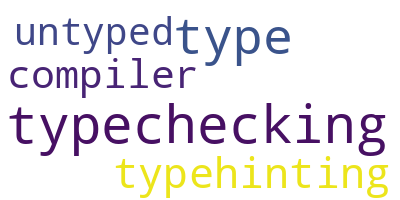
30-Days-Of-Python: Guide to Learning Python Programming Project
30 days of Python programming challenge is a step-by-step guide to learn the Python programming language in 30 days. This challenge may take more than100 days, follow your own pace. These videos may help too: https://www.youtube.com/channel/UC7PNRuno1rzYPb1xLa4yktw
https://github.com/Asabeneh/30-Days-Of-Python
pyright: Static Type Checker for Python Project
Static Type Checker for Python
https://github.com/microsoft/pyright
Python Is (Mostly) Made of Syntactic Sugar Article
“Programming languages are often made up of a (mostly) irreducible core, with lots of sugary constructs sprinkled on top—the syntactic sugar.” This article summarizes a lot of Brett Cannon’s recent work exploring just what is sugar in Python and what is fundamental.
https://lwn.net/Articles/942767/
Mojo, a Superset of Python Article
Mojo is a new programming language, which is a superset of Python. It aims to fix Python’s performance and deployment problems.
https://www.fast.ai/posts/2023-05-03-mojo-launch.html
How to Use Type Hints for Multiple Return Types in Python Article
In this tutorial, you’ll learn to specify multiple return types using type hints in Python. You’ll cover working with one or several pieces of data, defining type aliases, and type checking with a third-party static type checker tool.
https://realpython.com/python-type-hints-multiple-types/
Untyped Python: The Python That Was Article
This post by Armin is an opinion piece about the changes to Python over the years, particularly typing, and how that relates to other languages.
https://lucumr.pocoo.org/2023/12/1/the-python-that-was/
Pros and Cons of Dynamic Languages Article
This conversation is around Luke Plant’s excellent article Python’s “Disappointing” Superpowers that describes specific uses of Python’s dynamic capabilities that wouldn’t be possible in a static typed language.
https://news.ycombinator.com/item?id=34611969
Python Developers Survey 2022 Results Article
https://lp.jetbrains.com/python-developers-survey-2022/
Python 3.12: Static Typing Improvements Article
In this tutorial, you’ll see the new static typing features in Python 3.12. You’ll learn about the new syntax for type variables, making generics simpler to define. You’ll also see how @override lets you model inheritance and how you use typed dictionaries to annotate variable keyword arguments.
https://realpython.com/python312-typing/
How to Annotate Methods That Return self Article
In this tutorial, you’ll learn how to use the Self type hint in Python to annotate methods that return an instance of their own class. You’ll gain hands-on experience with type hints and annotations of methods that return an instance of their class, making your code more readable and maintainable.
https://realpython.com/python-type-self/
Why if TYPE_CHECKING? Article
“Typechecking is brittle yet important”, learn more about where it works and where it doesn’t and what that might mean for your code.
https://vickiboykis.com/2023/12/11/why-if-type_checking/
What’s the Zen of Python? Article
In this tutorial, you’ll be exploring the Zen of Python, a collection of nineteen guiding principles for writing idiomatic Python. You’ll find out how they originated and whether you should follow them. Along the way, you’ll uncover several inside jokes associated with this humorous poem.
https://realpython.com/zen-of-python/
Python Wins the NEC C&C Foundation Award Article
https://www.nec.com/en/press/202310/global_20231010_01.html
“Why Python Is Terrible” Article
https://news.ycombinator.com/item?id=36971851
Writing a C Compiler in 500 Lines of Python Article
This post details how to build a C compiler, step-by-step, using Python. A great intro to compilers. The target source is WASM, so learn a bit about that too.
https://vgel.me/posts/c500/
Why Mojo? Article
“A backstory and rationale for why we created the Mojo language.”
https://docs.modular.com/mojo/why-mojo.html
Ideas: Syntactic Sugar to Encourage Use of Named Arguments Article
https://discuss.python.org/t/syntactic-sugar-to-encourage-use-of-named-arguments/36217
How Many Python Core Devs Use Typing? Article
“How many old-school Python developers use type annotations?” This article dives into projects written by past and present core Python developers to see how often they use annotations in the wild.
https://blog.orsinium.dev/posts/py/core-devs-typing/
Idea: Return a NamedTuple Article
https://discuss.python.org/t/an-idea-to-allow-implicit-return-of-namedtuples/37546
What’s the Coolest Thing You’ve Done With Python? Article
https://www.reddit.com/r/Python/comments/17upt2f/whats_the_coolest_things_youve_done_with_python/
The Realest Python 😂 Article
https://twitter.com/realpython/status/1729541678975930382
Boring Python: Code Quality Article
How to build, deploy, and manage Python applications in as “boring” a way as possible in order to maximize stability and reliability. Also, read the Hacker News Discussion associated with the article.
https://www.b-list.org/weblog/2022/dec/19/boring-python-code-quality/
Type-Hinting Dataframes Article
This article demonstrates complete DataFrame type-hinting in Python, now available with generically defined containers in StaticFrame 2. In addition to usage in static analysis (with Pyright and Mypy), these type hints can be validated at runtime with an included decorator.
https://medium.com/towards-data-science/type-hinting-dataframes-for-static-analysis-and-runtime-validation-3dedd2df481d
Signed Distance Functions in 46 Lines of Python Article
A walkthrough of 46 lines of code that renders a 3D ASCII donut using signed distance functions.
https://vgel.me/posts/donut/
Why Did Python Win? Article
https://news.ycombinator.com/item?id=37308747
The Protocol Class Article
typing.Protocol enables type checking in a Java-esque interface like mechanism. Using it, you can declare that a duck-typed class conform to a specific protocol. Read on for details.
https://codebeez.nl/blogs/type-hinting-in-modern-python-the-protocol-class/
Obfuscated Python Competition Submissions Open Article
https://pyobfusc.com/
Microfeatures I’d Like to See in More Languages Article
Some language features are intrinsic to the language, others are syntactic sugar that could easily be borrowed in other programming languages. This opinion piece from Hillel highlights some features from more obscure languages that should be stolen by the mainstream. Two Python features he’d like to see in more languages are chained evaluations (2 <= x < 10) and numbers with separators (1000000 == 1_000_000).
https://buttondown.email/hillelwayne/archive/microfeatures-id-like-to-see-in-more-languages/
Type Hints: Passing Any for Unused Test Parameters Article
When you create a function to match an interface, it often needs to accept parameters that it doesn’t use. Once you introduce type hints, testing such functions can become a little irksome as Mypy requires all arguments to have the correct types. This article covers a technique to avoid that work.
https://adamj.eu/tech/2023/07/15/python-type-hints-pass-any-unused-parameters-tests/
Why Static Languages Suffer From Complexity Article
An extremely detailed, deep dive on how static type systems impact the consistency of languages. Hirrolot compares a variety of lesser known languages to see the consequences of their decisions. See also the associated Hacker News discussion.
https://hirrolot.github.io/posts/why-static-languages-suffer-from-complexity.html
Fighting With Python Type Hints Article
Miloslav wanted to properly type-hint a decorator. Turns out, it wasn’t the easiest thing to do. Read on for his solution.
https://pojman.cz/2023/typing-decorators/
Mojo: Head-to-Head With Python and Numba Article
This article covers a Mandelbrot-based benchmark of Python, variations of Numba, and the newly available Mojo. Although Mojo is fast it takes a lot more work than the author expected to translate Python to it, and with the right parameters Numba still beats it.
https://dev.to/maximsaplin/mojo-head-to-head-with-python-and-numba-5bn9
Running Untrusted Python Code Article
Andrew wanted to play with some untrusted Python. Read how he used seccomp and setrlimit to build a Python sandbox.
https://healeycodes.com/running-untrusted-python-code
Python Type Hints: pyastgrep Case Study Article
Previously, Luke wrote an article about what was involved in adding Type Hints to parsy. This follow-on article tackles the effort on a project with different challenges: pyastgrep.
https://lukeplant.me.uk/blog/posts/python-type-hints-pyastgrep-case-study/
Why Python Keeps Growing Article
https://news.ycombinator.com/item?id=35006777
Python Is Two Languages Now, and That’s Actually Great Article
Python’s addition of typing has created “two” languages: typed and untyped Python. Tin tells you why that’s a great thing.
https://threeofwands.com/python-is-two-languages-now-and-thats-actually-great/
What Learning APL Taught Me About Python Article
Sometimes learning a new language provides perspective in the ones you already know. Rodrigo picked up APL, and this article discusses what that taught him about Python.
https://mathspp.com/blog/what-learning-apl-taught-me-about-python
Gradual Soundness: Lessons From Static Python Article
A synopsis of a deep paper analyzing Static Python, a Python variant developed at Instagram to move from gradually-typed to statically-typed. Full paper available as PDF.
https://programming-journal.org/2023/7/2/
Functional Python, Part II: Dial M for Monoid Article
This article is about “commandeering techniques from richly typed, functional languages into Python for fun and profit.” The focus is on Typeclasses and continuation-passing style.
https://www.tweag.io/blog/2023-01-19-fp2-dial-m-for-monoid/
Three Python Trends in 2023 Article
An opinion piece on three trends likely to attract attention in the Python world in 2023: Python/Rust co-projects, web apps, and more typing. Read on for examples in each category.
https://blog.jerrycodes.com/python-trends-in-2023/
Python Proposal (Inspired by Lua) Article
https://hachyderm.io/@nedbat/110962461917632486
Elixir for Humans Who Know Python Article
Elixir is a functional based programming language with similarities to Ruby and Python. This article is an intro guide for programmers who know Python.
https://hibox.live/elixir-for-humans-who-know-python
Why I’m Still Using Python Article
This opinion piece from Eric talks about how he started with Python and why he’s continued on the journey. There is also an associated Hacker News Discussion.
https://www.mostlypython.com/p/why-im-still-using-python
Why Type Hinting Sucks! Article
https://old.reddit.com/r/Python/comments/10zdidm/why_type_hinting_sucks/
The Zen of Proverbs Article
Inspired by the Zen of Python and similar lists, this is list of Zen Proverbs for programming.
https://www.wagslane.dev/posts/zen-of-proverbs/
It Is Becoming Difficult for Me to Be Productive in Python Article
This discussion is based on Avinash’s article of the same name, where he describes his journey from type-less to typed languages and why he is finding it harder to refactor his Python.
https://lobste.rs/s/rpchmh/it_is_becoming_difficult_for_me_be
Python Quirks Article
A straight-out list of code snippets showing off some of the weird and unexpected behavior of your favorite language.
https://writing.peercy.net/p/python-quirks
Why Python Is Amazing Article
This opinion piece by Jos is a counter to the “its a terrible language” posts you come across once and a while. Read why Jos thinks Python is amazing.
https://josvisser.substack.com/p/why-python-is-amazing
2022 Real Python Tutorial & Video Course Wrap Up Article
“It’s been another year of changes at Real Python! The Real Python team has written, edited, curated, illustrated, and produced a mountain of Python material this year. We added some new members to the team, updated the site’s features, and created new styles of tutorials and video courses.”
https://realpython.com/podcasts/rpp/138/
The Contradictions in ‘The Zen of Python’ Article
This is a summary of Christopher Neugebauer’s talk at PyCascades reminding attendees how foolish consistency can be a hobgoblin to productivity.
https://thenewstack.io/the-contradictions-in-the-zen-of-python/
Ode to Ptyon Article
A lighthearted poem about a certain well-known programming language.
https://medium.com/@petefison/ode-to-python-7e273ae1762b
Breaking the Snake: How Python Went From 2 to 3 Article
This discussion spawned from Diego Crespo’s article of the same name talks about the transition that somehow everyone is still interested in talking about.
https://news.ycombinator.com/item?id=34439876
python-skyfield: Elegant Astronomy for Python Project
Elegant astronomy for Python
https://github.com/skyfielders/python-skyfield/
CausalPy: Causal Inference in Quasi-Experimental Settings Project
A Python package for causal inference in quasi-experimental settings
https://github.com/pymc-labs/CausalPy
tragic-methods: Collection of Programming Quirks Project Started in 2023
A collection of script depicting the strange quirks of programming languages.
https://github.com/neemspees/tragic-methods
symbex: Search Python for Symbols to Output to an LLM Project Started in 2023
Find the Python code for specified symbols
https://github.com/simonw/symbex