The biggest Python topics of 2023 ›
Working Efficiently with Lists and Iterables in Python
Exploring Pythonic List Handling and Iteration: This topic delves into the effective management of lists in Python, including the utilization of list comprehensions, iterators, and iterable objects. It highlights best practices, common pitfalls, and efficient approaches for manipulating lists and sub-list structures. By employing Pythonic techniques and iterators, developers can streamline data processing tasks and enhance code readability.
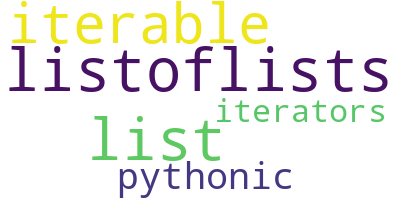
Python’s list: A Deep Dive With Examples Article
In this tutorial, you’ll dive deep into Python’s lists. You’ll learn how to create them, update their content, populate and grow them, and more. Along the way, you’ll code practical examples that will help you strengthen your skills with this fundamental data type in Python.
https://realpython.com/python-list/
Python’s tuple Data Type: A Deep Dive With Examples Article
In Python, a tuple is a built-in data type that allows you to create immutable sequences of values. The values or items in a tuple can be of any type. This makes tuples pretty useful in those situations where you need to store heterogeneous data, like that in a database record, for example.
https://realpython.com/python-tuple/
Using Python’s min() and max() Article
In this video course, you’ll learn how to use Python’s built-in min() and max() functions to find the smallest and largest values. You’ll also learn how to modify their standard behavior by providing a suitable key function. Finally, you’ll code a few practical examples of using min() and max().
https://realpython.com/courses/python-min-max/
Python’s Mutable vs Immutable Types: What’s the Difference? Article
In this tutorial, you’ll learn how Python mutable and immutable data types work internally and how you can take advantage of mutability or immutability to power your code.
https://realpython.com/python-mutable-vs-immutable-types/
Ideas: Allow Comprehension Syntax in for Loop Article
https://discuss.python.org/t/allow-comprehension-syntax-in-loop-header-of-for-loop/25864
Filtering Iterables With Python Article
In this video course, you’ll learn how Python’s filter() works and how to use it effectively in your programs. You’ll also learn how to use list comprehension and generator expressions to replace filter() and make your code more Pythonic.
https://realpython.com/courses/python-filter-function/
Everything You Can Do With Python’s bisect Article
Learn how to optimize search and keep your data sorted in Python with the bisect module
https://martinheinz.dev/blog/106
5 Ways to Flatten a List of Lists Article
Flattening a list-of-lists is the process of taking each of the inner lists’ contents and putting them together in a single list. There are several ways of attacking this problem in Python, and this article shows 5 methods ranked from worst to best.
https://mathspp.com/blog/5-ways-to-flatten-a-list-of-lists
Getting the First Match From a Python List or Iterable Article
In this video course, you’ll learn about the best ways to get the first match from a Python list or iterable. You’ll look into two different strategies, for loops and generators, and compare their performance. Then you’ll finish up by creating a reusable function for all your first matching needs.
https://realpython.com/courses/python-first-match/
Text Editor Data Structures Article
This article details the different ways a text editor represents text in memory and the advantages of each. Unfortunately, the examples aren’t in Python, but there is plenty CS stuff to dig into here.
https://cdacamar.github.io/data%20structures/algorithms/benchmarking/text%20editors/c++/editor-data-structures/
Efficient String Concatenation in Python Article
In this tutorial, you’ll learn how to concatenate strings in Python. You’ll use different tools and techniques for string concatenation, including the concatenation operators and the .join() method. You’ll also explore other tools that can also be handy for string concatenation in Python.
https://realpython.com/python-string-concatenation/
How to Write Efficient Python Code: A Tutorial for Beginners Article
Are you a programmer looking to get better at Python? Learn some of Python’s features that’ll help you write more elegant and Pythonic code.
https://www.kdnuggets.com/how-to-write-efficient-python-code-a-tutorial-for-beginners
Why You Should Not Overuse List Comprehensions in Python Article
List comprehensions in Python are super helpful one-liners. But if overused, they can make your code a pain to maintain. Here’s why.
https://www.kdnuggets.com/why-you-should-not-overuse-list-comprehensions-in-python
One Liners Python Edition Article
A collection of single-line Python snippets that cover common tasks in programming. Includes removing duplicates from lists, reversing strings, finding the most common element in a list, and much more.
https://muhammadraza.me/2023/python-oneliners
How I Added C-Style for-Loops to Python Article
Ever want a C-style for-loop in Python? No? Well you can have one anyway. See how Tushar implemented with for (i := var(0), i < 10, i + 2):
https://tushar.lol/post/cursed-for/
Iterators and Iterables in Python: Run Efficient Iterations Article
In this tutorial, you’ll learn what iterators and iterables are in Python. You’ll learn how they differ and when to use them in your code. You’ll also learn how to create your own iterators and iterables to make data processing more efficient.
https://realpython.com/python-iterators-iterables/
Make Each Line Count, Keeping Things Simple in Python Article
Simplicity is hard. This article talks briefly about how you approach coding while keeping things simple.
https://pybit.es/articles/make-each-line-count-keeping-things-simple-in-python/
Does Elegance Matter? Article
In this article, the author explains why he thinks that elegance should be a fundamental driver when you are writing (Python) code, and gives a few tips on how to write elegant code.
https://mathspp.com/blog/pydonts/does-elegance-matter
How to Flatten a List of Lists in Python Article
In this tutorial, you’ll learn how to flatten a list of lists in Python. You’ll use different tools and techniques to accomplish this task. First, you’ll use a loop along with the .extend() method of list. Then you’ll explore other tools, including reduce(), sum(), itertools.chain(), and more.
https://realpython.com/python-flatten-list/
Counting Occurrences in Python With collections.Counter Article
Python’s collections.Counter objects are helpful for counting occurrences of iterable items. They’re especially helpful when paired with generator expressions.
https://www.pythonmorsels.com/using-counter/
How to Split a Python List or Iterable Into Chunks Article
This tutorial provides an overview of how to split a Python list into chunks. You’ll learn several ways of breaking a list into smaller pieces using the standard library, third-party libraries, and custom code. You’ll also split multidimensional data to synthesize an image with parallel processing.
https://realpython.com/how-to-split-a-python-list-into-chunks/
Python’s list Constructor: How to Use It and How Not To Article
When should you use the built-in list(…) function in Python? And when shouldn’t you? The list constructor is both underused and overused in Python.
https://www.pythonmorsels.com/using-list/
De-Duplicating a List While Maintaining Order Article
There’s more than one way deduplicate an iterable in Python. Which approach you take will depend on whether you care about the order of your items.
https://www.pythonmorsels.com/deduplicate-lists/
Summing Values the Pythonic Way With sum() Article
In this video course, you’ll learn how to use Python’s sum() function to add numeric values together. You’ll also learn how to concatenate sequences, such as lists and tuples, using sum().
https://realpython.com/courses/python-sum-function/
panflute: Filters for Pandoc Project
An Pythonic alternative to John MacFarlane's pandocfilters, with extra helper functions
https://github.com/sergiocorreia/panflute